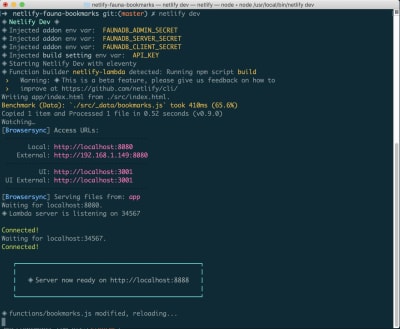
This allows you to make simpler use cases more streamlined while still providing escape hatches for more complex scenarios. This method is very simple, all we do is assign the value "unknown" directly to the parameter, it will only be changed if you call the function and specify a new value for the parameter. It has a three operands. Today we will show you the list of possible ways to handle the default parameter value in JavaScript. For any inquiries, contact us at [emailprotected]. Create Javascript functions with optional parameters that assume a default value if no argument is given. Instead of specifying optional parameters in the function signature let's update the function to accept a parameter called options. This can be remedied in part by using the composable functions discussed previously. This is because there is no way to specify optional parameters by name, the orders matters and is required. Receive the latest articles straight to your inbox. For a list of trademarks of the OpenJS Foundation, please see our Trademark Policy and Trademark List. We strive to have a friendly environment for developers to engage and help each other out. Save my name, email, and website in this browser for the next time I comment. All rights reserved. JS PRO TIP: In Math.pow(base, power) if power is 0 or any other "falsy" value (except NaN) the result will always be 1. However, you will sometimes run into situations where you want to provide a default value for a parameter or take a variable number of parameters.
Object spreading is a helpful new feature which can help with the problem described above. Code Premix 2022. If it is set, we simply re-assign its own value to itself, if it is undefined then we assign it a default value of "unknown". Changing the 7th optional parameters means specifying optional parameters 1 through 6 even though you wanted to use the defaults all along! This method works very well as it is cross-browser and is a relatively simple way of achieving optional parameters with default values like in other programming languages that have it built in. This does have the potential to mess with the rest of your function as it could be relying on the parameter being of a certain type such as a string or integer. :). But, you may want all number cells right-aligned to make it easier to scan. The optional parameter is not the undefined value in this case - the last parameter is the undefined one. Trademarks and logos not indicated on the list of OpenJS Foundation trademarks are trademarks or registered trademarks of their respective holders. Default Parameters in JavaScript, Understanding Default Parameters in Javascript with examples, Set a default parameter value for a JavaScript function, es6 default parameters object, javascript multiple optional parameters, javascript default value if undefined, javascript function optional parameter default value. It's not hard to imagine a scenario where there are many default parameters that could be updated this way in a real-world application. |, With additional type check (safer option), Expressions can also be used as default values, Replace multiple strings using Split and Join functions, Difference between var, let and const in JavaScript, How to Verify that an URL is an Image URL using JavaScript, Method of promises in JavaScript with example, How to wait for all images to load in JavaScript, How to push code to GitHub using git bash, How to convert a string to an integer in JavaScript, How to use Map, Filter, and Reduce in JavaScript. Sometimes people use the idiom: optionalParameter = optionalParameter || defaultValue. This is generally not going to be your intention and should be avoided or handled with extra caution. So a better way to do this is by explicitly checking that the optional parameter is undefined. See something you don't agree with or feel could be improved? All without having to specify a foodType! One of the features added to ES2018 is object spreading with object literals. The example shows you how to do that: Copyright OpenJS Foundation and Node.js contributors. Its also known as a ternary operator. // { name: 'Tom', color: 'grey', foodType: 'dry', favoriteToy: 'yarn' }, // { title: 'New Cell', prop: { itemA: 1 }, itemB: 2 }, // { title: 'New Cell', prop: { itemA: 'ABCD' }, itemB: 2 }. This can have some undesirable behavior when they pass values that are equal to false such as false, 0, and "". Spreading allows developers to copy an object and spread it into another object creating a copy of the new and old object merged together. This case is also valid for modern JavaScript(ES6/ES2015). Here we used the 0 as default value in parameter and also you can use getDefaultNumber() function as default values in parameter of another function. It means that if you dont pass the arguments to the function, its parameters will have the default values of undefined. Or just chat to another develop who can help you. All of that flexibility comes at a cost and optional parameters are often used to guide you in the right direction. In JavaScript, a parameter has a default value of undefined. Here we use an OR operator to re-assign the same value to the parameter variable "user_nickname" as long as it is not undefined. Here we used the conditional operator (? It can be difficult for users of the function to know what the available options are compared to explicitly using function parameters. We care about privacy and will never spam you. This is great for setting default values in a function and then allowing callers of the function to override them with other values. Join our community and fine more people with your skills! So we dont need to use the OR (||) operator to assign default value in variable. This method is the shortest way of achieving the desired result. and will halt your Javascript code resulting in your site not functioning properly. In this method we use a ternary operator to check whether the type of the parameter variable "user_nickname" is undefined which would mean it has not been set. This can be an issue if either of these is a valid input in your function.
Better still we can compose them together to create a more friendly API for users. There are several ways to accomplish this task in Javascript.
The OpenJS Foundation | Terms of Use | Privacy Policy | Bylaws | Code of Conduct | Trademark Policy | Trademark List | Cookie Policy. Any JavaScript expressions can also be used as default values for function parameters. This is fine and the spread operator will not throw an error. This is done by checking if the last parameter is undefined and setting it to a default value if it is. We'll take a look at how we can use objects and the spread operator to provide good default values while still allowing for overrides in a much simpler way. Privacy Policy. Even though a: 2 is created directly in the data object it is overwritten by the newData that is being spread into the object. We are not simply proficient at writing blog post, were excellent at explaining the way of learning which response to developers. Read our Users will need to dig into the function to see what options are available and how they should be set as it is not immediately obvious. Usually a function will take a set number of parameters, and require that all of them be present before it can be executed successfully. If you are not sure about the value type of the variable then you can use ternary operator to reduce the bug in code. The first idiom is giving a default value for the last parameter.