How to Deploy Contract From NodeJS using Web3? So we need to use await to get the actual value. Unlike Array.from, mapfn may be an async function.) The main difference with the previous one is that Promise.all() executes all asynchronous operations in parallel.
Sr Software Engineer | AWS Certified Solution Architect | Auth0 Ambassador | Blogger | Stackoverflower. What is the difference between null and undefined in JavaScript? .
For further actions, you may consider blocking this person and/or reporting abuse. A sync function can work in an async environment, but an async one can not be converted to work in a synchronous way. Let's keep this post just for quick cheatsheets . With you every step of your journey.
and only accessible to Afif Sohaili.
In the future, standardizing an async spread operator (like [ 0, await v ]) Lets have look at our scenario. but the above code is also valid, and shows how to use promises in Array.map(), which is the point of this tutorial. If its mapping callback throws an error when given an input value, then its promise will reject with that error. for dumping the entirety of an async iterator If its inputs iterator throws an error while yielding a value, then its promise will reject with that error. If the array-like objects elements are promises, then each accessed promise is awaited before its value is added to the new array. How to resolve 'node' is not recognized as an internal or external command error after installing Node.js ? I'm new to javascript but not new to programming. depends on whether Object gets fromEntriesAsync. Yep, definitely a great library for Promises. I publish new posts every week or two about JavaScript and Node.js, including dealing with asynchronous scenarios. For example, a string can be doubled without any asynchronicity involved: If the function is async, its also easy for a single item using await. For example, to calculate the SHA-256 hash, Javascript provides a digest() async function: This looks almost identical to the previous call, the only difference is an await. NOTE: Please remember that the Promise.All() will return a single Promise. Most often than not, this is my preferred solution as it's quite uncommon to want promises to execute sequentially. That way, if you have a requirement where you need to ensure each function resolves in order, you will be able to and won't be scratching your head trying to figure out why the functions are resolving out of order And if you need them to be concurrent, you'll be able to do so and get them to resolve faster than if you were doing it in series. The new async/await is an immensely useful language feature that hides the complexity of asynchronous code and makes it look like its working in a synchronous way.
The code example below does not look complicated at all, just a few awaits here and there. I write articles and books to help you be that expert. Of course, if you want the return values of these async functions, you could do: Quick note: this post is just covering the "happy path" for now (i.e. How to read and write Excel file in Node.js ? Still keeping it here if you're interested. Only minor formatting changes have been made to the status-quo examples. Last Updated Jul 08 2021. Learn the basics of CloudFront configuration and: Learn how to use async functions in Javascript from our free email-based course, Learn the basics of serverless computing on AWS from our free email-based course. Array.fromAsyncs valid inputs are a superset of Array.froms valid inputs. Sorry I hadn't come and check this often enough and missed it. You can apply the above way to solve these kinds of issues in all array methods and other functions that do not handle async callbacks with await. I just felt like most of the time what I need with Promises are already available in ES6+. So the output result will be a Promise. From the first steps with asynchronous programming in Javascript to an intricate understanding of how Promises and async functions work. How to return an error if response not received in 1 minute in Node.js ? later in this explainer. Posted on Jun 14, 2019 We have to call the async function from another function which can be asynchronous or synchronous (We can pass the array or choose to declare the array in the async function itself) and then return the array from the async function. How to get the days between 2 dates in JavaScript, How to redirect to another web page using JavaScript, How to replace white space inside a string in JavaScript, How to return multiple values from a function in JavaScript. Once we have that, we can iterate over each collection of async functions and execute them in parallel, and use Promise.all to wait for each of those functions to complete. Next time you need to remember how to execute the two types, reference this post. For a more realistic scenario, querying a database is inherently async as it needs to make network connections. SLIIT FOSS Community is a team of volunteers who believe in the usage of Free/Open Source Software (FOSS). Because we are waiting for the function to convert it so we can print it in the console. I have to be honest I haven't used for loops in a while so it didn't really occur to me. Array.from has become one of Arrays If anything wrong happens while executing the try block statements, then a catch block is used to handle the exception. by various developers, asking how to convert async iterators to arrays. I am pretty sure it might be so confusing.
But without async functions, you can not use await and provide a result later which is required for things like reading a database, making network connections, reading files, and a whole bunch of other things. Given a task that requires writing software, an expert provides better and more reliable solutions. Let's keep in touch and: Thank you for your interest in the book! How to connect to Telnet server from Node.js ?
That's interesting. For executing concurrently, I recommend using Promise.all(). What are the advantages of synchronous function over asynchronous function in Node.js ? How to install the previous version of node.js and npm ?
If its input throws an error while creating its async or sync iterator, then its promise will reject with that error. Array.fromAsync has two optional parameters. (A formal draft specification is available.). This makes use of the same concept in #3, where we have an array of promises resolved sequentially, combined with a two-dimensional array of promises and the use of Promise.all. How to Create a Linked List in JavaScript. Its not just adding an async before the function passed to Array.reduce and it will magically work correctly. (Values that are not promises are also awaited for one microtick to prevent Zalgo.) (Several real-world examples are included in a following section.). The following built-ins also resemble Array.from: We are deferring any async versions of these methods to future proposals. Come write articles for us and get featured, Learn and code with the best industry experts. A co-champion of iterable-helpers seems to agree that we should have both or that we should prefer Array.fromAsync: Yes, you can abstract those however you'd like to make it more readable, and functions are something you can toss around in JavaScript.
It will print output as you expected.
In Java, for example, you can create a new Thread and then it does not matter if some commands block it. Im Just Trying to Fetch SOMETHINGANYTHING Right Now. The map() method returned an Array of Promises instead of an Array of Strings. Their exclusion would be surprising to developers who are already used to Array.from. I've got another post planned for soon that will deal with more robust error handling. As for loops are a generic tool, they can be used for all kinds of requirements when working with collections. The return value is still a promise that will resolve to an array. The job of Promise.all() is taking an Array of Promises and return values after all the promises are resolved. This becomes overly apparent when you do too much computation and it freezes the page which is when Chrome shows the Page unresponsive popup. Published Oct 11 2018, Asynchronous operations seem to trip up a lot of developers. Sign up below to get all my new posts on these topics: // call each function to get returned Promise, // 2s (roughly) - 'first', 'second', 'third'. I write about technology to deepen my knowledge and also to help others solve problems. I remembered why its better for a buildable structure to consume an iterable than for an iterable to consume a buildable protocol. If we pass async functions to forEach, we have no way of retrieving the returned promise to do anything useful with it. Templates let you quickly answer FAQs or store snippets for re-use. And async/await is another language feature that makes it a lot easier to write asynchronous code. Such functionality would be useful
You may say that we passed an async method inside the map(), So we need to use await in front of it like below. A function returning a promise to be applied to each of the above. How to Convert CSV to JSON file having Comma Separated values in Node.js ? But for collections, handling asynchronicity becomes different. Promise.all() provides another option for asynchronous loops over arrays. It will become hidden in your post, but will still be visible via the comment's permalink. We already have Array.from. However, no similar functionality exists for async iterators. How to Rewrite promise-based applications to Async/Await in Node.js ? Remember, the difference between Array.prototype.map and Array.prototype.forEach is that the latter does not return the result of each iteration. Because Still, beginners are struggling to understand this concept. By signing up to the free chapters you'll receive 4 emails, each with a different part of the book. acknowledge that you have read and understood our, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam. The easiest way to run an array of async/await functions in series is to use forof. of the Array built-in class, with one required argument The Tuple constructor, too, would probably need an fromAsync method. The MDN docs would be a good place to start, and CSS-Tricks has a good article on it as well. Get access to ad-free content, doubt assistance and more!
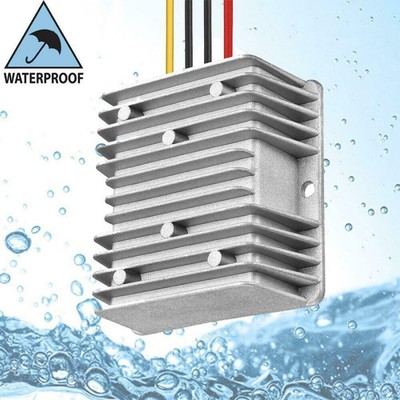