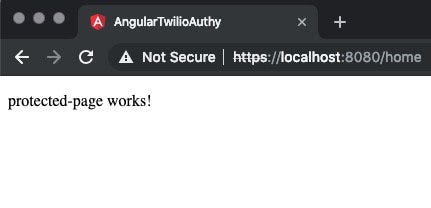

Many operations in JavaScript and on the web can result in data streams. Finally, don't forget to pay special attention if you're developing commercial Angular apps that contain sensitive logic.
To build it, we'll use the fromEvent method, which we'll import like this: Now that we can build Observable streams with the fromEvent method, let's actually build one. You can protect them against code theft, tampering, and reverse engineering by following our guide. Setting up permissions based on user roles is a common use case in web apps. We can also emit data structures into observable streams ourselves.
Next, let's see an example of handling an HTTP request with an Observable in Angular 8. This "data over time" concept is great when working with asynchronous, event-based applications. Feel free to test out other sources of API data, or build your own and serve it to your Angular app. Finally, to test what running the Observer.next() method will be like, let's connect to a different, open-source API.
In this tutorial, you'll learn how to implement an Angular file upload mechanism using both Angular and Node.js.
What you'll do to watch a TV show online can be summed up in a few steps: Note: in this example, you can keep watching if there are episodes available.
the streaming show is the Observable stream; the person watching the show is the Observer; the act of pressing the play button is known as subscribing; the act of pressing the stop button is called unsubscribing; primitive values such as strings or numbers; click events (or any other event for that matter! If we choose not to pass a handler for either one of these notifications, the Observer will simply ignore them. Sometimes, the data source is referred to as the Producer, because it supplies the data stream to the Observable.

Hopefully, both examples have illustrated the possibilities of using Observables. ). You are the Observer and the show that's streaming is the Observable. And that sums up our second Observables example in Angular 8!
An observable is like a function that returns a data stream, either synchronously (at once), or asynchronously (over time). I also write technical articles. RxJS is a library that makes it possible for us to work with these streams of data in JavaScript. You can easily find free APIs in this GitHub list. In RxJS, the Observer is an Object with 3 built-in methods: next(), error(), and complete(). Feel free to see more info in the official documentation. Once the entire show stream is complete, you get the complete notification so you know there are no more episodes to watch and the player stops on its own. Great, whenever a new episode is emitted, besides watching the stream, we're also having a piece of pizza. That's why we'll add a subscription right inside our component's constructor: We're taking care of each of the three built-in scenarios: next(), error(), and complete(). Visit the streaming service and locate the show to watch; The show starts to arrive over time, episode by episode; You want to keep binge-watching but you must go to work so you click the stop button.
What kind of data can be emitted from an Observable stream? Thus, the Observable delivers (emits) some values, and the Observer knows what to do with them. For this, we'll use a simple app hosted on Stackblitz. I agree to receive these emails and accept the. This is known as handling the next condition. Here's a real-world metaphor that we can use to get our head around the concept of observables.
Being the Observer, you can get three different notifications: next, error, and complete. If we need to do something each time an episode is emitted, we pass that as a parameter to the next() method. When there are no episodes available, you will keep listening for new episodes whenever they appear unless the streaming service has marked the series as over, as well explain below. If an error occurs, such as our internet connection breaking, we can handle the error condition like this: When the stream is complete, we can read a book, like this: An Observer is like a person that watches the data stream and based on what emits from the stream (i.e based on notifications from the stream) does some predefined action accordingly.
We'll make an Observable from mousemove events in the window object: However, even though the data is there, we can't receive it until we subscribe to the Observable stream. Let's say you want to watch a TV show from any of the available online show-streaming services.
Whenever the next episode comes through the data stream, you get the next notification. Thus, whenever a new episode is emitted, the next() method gets called. I'm a senior software developer and published author of several programming books.
In RxJS parlance, we say that each episode is emitted from the stream.
With functions, we control when we get the value out (we pull the value out by calling the function i.e making the code inside the function run and return a value); With observables, we only listen to the Observable, which pushes the values to us; thus, the Observable is in control. Note that these three are passed in as ES6 anonymous functions, with function parameters given some custom names.