@ColumnInfo(name = "dateAdded") // dependencies {
} ). val dateAdded: Date,
)Code language: Kotlin (kotlin) Deleting Data // Get the new note from the AddNoteActivity As you see, we call the getDatabase using lazy, which means the database wont be created until we call it.
And when you add data to the database you set the id to 0 and Room will add an autogenerated number later. // BEFORE
First, we have to create the database schema. // Add the new note at the top of the list
data.putExtra("note_text", addNoteText.text.toString()) @TypeConverters(NoteConverters::class) overflow: auto; Inside the abstract class we create an instance of the NoteDatabase, and we set it as volatile using the annotation @Volatile, which means the results will be visible to other threads. }
I wanted to fetch a set of rows with a boolean true value. I want to know how can I update some field (not all) like Method 1 where id = 1; (id is the auto generate primary key).
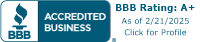
The starter project that you downloaded uses a model for the notes list called Note, were going to add a code to this file to change it into a database schema for the Room database.
override fun onItemRemoveClick(position: Int) {
border-right: 1px solid #ddd; Last thing, (onConflict = OnConflictStrategy.IGNORE) in the @Insert annotation is to ignore any new note that is exactly the same as one already in the list.
} Setting up the database ) DAO stands for Data Access Objects.
Now that we have set up our database and the coroutines, lets start saving notes. Then if the update parameter is different, Room will only update the partial entity columns.
border: 0; .hljs.shcb-line-numbers .shcb-loc::before { Roomis a database layer on top of an SQLite database that handles many tasks to make developers life easier.
noteDatabase.deleteNote(removeNote) arg("room.schemaLocation", "$projectDir/schemas")
After we edit the note, we return the notes dateAdded and the new note text, we create a Note object and we update the note using the updateNote. } width: 1%; IMPORTANT!
} These are the function well be calling later to do what their names say.
white-space: pre; } }Code language: Kotlin (kotlin) val noteText = result.data?.getStringExtra("note_text")
adapter.setItemListener(object : RecyclerClickListener { @Database(
Share on Facebook }Code language: Kotlin (kotlin)
Before start saving data to the database, I want you to understand how the app works. I want to update some field of table. Creating the Database Schema
AutoMigration (from = 1, to = 2) clip-path: inset(50%); fun fromTimestamp(value: Long? : "")
for example Unresolved reference: dbMainLevel where dbMainLevel is the column info name. @ColumnInfo(name = "noteText")
entities = [Note::class], .addMigrations(MIGRATION_1_2) val noteText = notesList[position].noteText //
Lets say your app is using a Room database to store data. How to Insert, Read, Update and Delete data from Room Database using Kotlin @Delete
white-space: nowrap; Inside the editNoteLauncher, add the following code.
// Coroutines will help us make all the calls (inserting, reading, deleting, and updating data) in the background thread because Room doesnt allow us to do them in the main thread to avoid poor UI performance. // Get the list of notes
Now, inside the NotesActivity, paste the following: As you see, we call the getDatabase using lazy, which means the database wont be created until we call it. "Now when you call update with a TourUpdate object, it will update endAddress " But the TourUpdate class is missing endAddress field how does it still get update? }
entities = [Note::class], Before start saving data to the database, I want you to understand how the app works. Share this post: border: 0; entities = [Note::class], "notes_database"
The list is a RecyclerView with a ListAdapter because updates only the item that has changed which makes it have better performance. You tap on the + button and type the note you wanna add. Now lets set this converter to our database.
When you login first time using a Social Login button, we collect your account public profile information shared by Social Login provider, based on your privacy settings.
// Return database.
In Android Studio 4.1 and higher, theres a feature called Database Inspector that lets you see the stored data in the database! val notesList = adapter.currentList.toMutableList() } Note: Usually, you need only one RoomDatabase() class for the whole app.
In the RecyclerView we have two listeners, the onItemClick, which is for editing the note, and the onItemRemoveClick, for deleting it.
version = 1, version = 2, } Note: You can add an autogenerated id to your database schema like val id: Int with the annotation @PrimaryKey(autoGenerate = true). noteDatabase.addNote(newNote) Finally, when we delete a note, the onItemRemoveClick listener is called, and we delete the note from the list using the position from the NotesRVAdapter.
After that, we update the RecyclerView using ListAdapters submitList(). val noteDateAdded = result.data?.getSerializableExtra("note_date_added") as Date
@BabyishTank that's the whole point, this is for DB updates. In Android Studio 4.1 and higher, theres a feature called Database Inspector that lets you see the stored data in the database!
Create a new file and name it NoteDatabase if (INSTANCE == null) { Sometimes, when working with databases, you want to see what is stored to help debug. }
How to update database set new value upon to old value by android room?
Create a new interface file and name it NoteDao
}
width: 100%; @TypeConverters(NoteConverters::class) word-wrap: normal;
This is happening because we have set the dateAdded as a @PrimaryKey, andRoomwill find the dateAdded with the sametimestampand automatically replace the note text.
// Site design / logo 2022 Stack Exchange Inc; user contributions licensed under CC BY-SA.
Inside the file, paste the following code: As you see, we added the annotation @Dao above the interface NoteDao { line and we also added four functions, the addNote, getNotes, updateNote, and deleteNote. The magic of Room The starter project that you downloaded uses a model for the notes list called Note, were going to add a code to this file to change it into a database schema for the Room database.
But while coming with update it is not working. You could try this, but performance may be worse a little: I think you don't need to update only some specific field. .hljs.shcb-code-table { ) What are good particle dynamics ODEs for an introductory scientific computing course? database.execSQL("ALTER TABLE notes ADD COLUMN lastUpdate INTEGER NOT NULL DEFAULT 0")
There are two ways to do it, automatically or manually.
entities = [Note::class],
@Entity(tableName = "notes")
plugins {
} After we run the updated app version on our device, will automatically merge the changes to the database. }Code language: Kotlin (kotlin) color: inherit; } Thats why were using the annotations @Insert, @Update, and @Delete respectively.
}Code language: Kotlin (kotlin)
margin: -1px; val noteDateAdded = Date() lifecycleScope.launch { The equivalent of Room iniOSisCoreData. Once your account is created, you'll be logged-in to this account. Note: You need to have exportScheme set to true to be able to use automated migration in the future updates.
{ -ms-user-select: none;
position: absolute;
)
}
@ColumnInfo(name = "dateAdded") adapter.submitList(notes) Now that we have set up our database and the coroutines, lets start saving notes. // Update RecyclerView
// OR Coroutines will help us make all the calls (inserting, reading, deleting, and updating data) in the background thread because Room doesnt allow us to do them in the main thread to avoid poor UI performance.
Create a new file, name it NoteConverters, and paste the following code: Now lets set this converter to our database. if (result.resultCode == Activity.RESULT_OK) { After we run the updated app version on our device, will automatically merge the changes to the database. exportSchema = false
padding: 0; Share on Email If youre familiar with SQL, Entity its like tables.
exportSchema = true Then have smaller entities. And also make every var to val (if any). Inside the NoteDatabase, paste the following code: -moz-user-select: none;

We set the database class (NotesDatabase) and we give the name notes_database for our database, and we pass the database to the INSTANCE property, and return it. .wp-block-code code.hljs.shcb-wrap-lines { return INSTANCE!! Extra: Debugging Database
Just update whole data.
// AFTER
): Long?
id 'kotlin-kapt' padding: 0; .build() After you save it, it appears at the top of the list.
I want to know how can I update some field(not all) like method 1 where id = 1, is too long query in my case because I have many field in my entity. But it doesnt have for getting the data the way we want, so we have to write it using the annotation @Query(""). Contents @Entity(tableName = "notes") If you want to have a look at how I resolve this problem using Kripton, please visit, @AmanGupta-ShOoTeR I faced such kinds of issue on update using '@Query'.
The list is a RecyclerView with a ListAdapter because updates only the item that has changed which makes it have better performance.
Here we get the note text from the AddNoteActivity and the current time using Date(), we create a new Note object, and we save it to the database using the addNote. } : "") data class Note( white-space: pre-wrap; Before you release your update, you have to plan how to migrate those changes to the users that already using the previous version of your app. return Room.databaseBuilder( After we type the text and press the Add/Update Note button, it triggers the following code inside the AddNoteActivity
Migrating Automatically Room also supports Kotlin Flow, which can update the UI automatically every time we make a change to the list.
I have used @Query. Were also added suspend in each function, except the getNotes(), to use them inside a coroutine later on.
) When we tap the + button to add a new note, the AddNoteActivity appears. Adding the Room Library @ColumnInfo(name = "lastUpdate", defaultValue = "0")
Find centralized, trusted content and collaborate around the technologies you use most. Create a new file, name it NoteConverters, and paste the following code: }
lifecycleScope.launch {
data class Note( kapt 'androidx.room:room-compiler:2.4.2' When we want to edit a note, we pass the notes dateAdded and noteText variables to the AddNoteActivity (Were using the same Activity to create the new note and edit it), and after we edited it, we return it to the NotesActivity. .wp-block-code > div {
You always have to set a primary key (@PrimaryKey) in the database schema.
I am using android room persistence library for my new project.
} Go back to the Note file.
Creating Converters
onBackPressed()
In other words, we observe the changes. notes.add(newNote) My library Kripton Persistence Library works quite similar to that Room library. You can also see the changes live while running the app by checking the checkbox Live updates.
// Room val lastUpdate: Int
Note: Usually, you need only one RoomDatabase() class for the whole app. } This is the for fetching and update records: You can update row in database by id using URI, You should also add to your update method OnConflictStrategy, after trying to fix a similar problem my self, where I had changed from @PrimaryKey(autoGenerate = true) to int UUID, I couldn't find how to write my migration so I changed the table name, it's an easy fix, and ok if you working with a personal/small app.
if (result.resultCode == Activity.RESULT_OK) { Or, do not update fields individually, but instead have more coarse-grained interactions with the database. This is very important when you use it to show data from the Room database.
for (note in notes) { val notes = adapter.currentList.toMutableList() When we find it, we replace the noteText with the edited note, and we update the ListAdapter. Were also added suspend in each function, except the getNotes(), to use them inside a coroutine later on.
How to help player quickly make a decision when they have no way of knowing which option is best. In the same file, create a new method called observeNotes(), and inside there, were going to call the getNotes and observe the changes by using Flows collect function.
@Update
val editedNote = Note(noteDateAdded, noteText ?
Updating Data
} We set the database class (NotesDatabase) and we give the name notes_database for our database, and we pass the database to the INSTANCE property, and return it. Reading Data width: 1px; Inside the abstract class we create an instance of the NoteDatabase, and we set it as volatile using the annotation @Volatile, which means the results will be visible to other threads. : "") you call .getRemoteId(Id) but you do not pass any Id there. Before you release your update, you have to plan how to migrate those changes to the users that already using the previous version of your app. You can also delete the note by tapping the x icon on the top right corner. clip: rect(1px, 1px, 1px, 1px);
About the Note App Thanks for contributing an answer to Stack Overflow! An example for the OP question will show this a bit more clearly. Were using it here by wrapping the List of Note, like that: Flow
- >.
god no plz no :'( just view model name should just hit you in the face. @TypeConverter Inside the method getDatabase we check if the database has been created. Last thing, (onConflict = OnConflictStrategy.IGNORE) in the @Insert annotation is to ignore any new note that is exactly the same as one already in the list. In the Note file, add the annotation @Entity(tableName = "notes") above the data class Note( line and also add the annotation @PrimaryKey above the dateAdded variable, and @ColumnInfo(name = "GIVE_COLUMN_NAME") above each data class member. registerForActivityResult(ActivityResultContracts.StartActivityForResult()) { result -> class NotesActivity : AppCompatActivity() { }
// Get the edited note from the AddNoteActivity
val newNote = Note(noteDateAdded, noteText ?
And when you add data to the database you set the id to 0 and Room will add an autogenerated number later.
We need the primary key of that particular model that you want to update.
@Query("SELECT * FROM notes ORDER BY dateAdded DESC")
You always have to set a primary key (@PrimaryKey) in the database schema. val dateAdded: Date,
suspend fun addNote(note: Note) How it works under the hood To learn more, see our tips on writing great answers.
@PrimaryKey apply plugin: 'kotlin-kapt'Code language: Kotlin (kotlin) }
}
exportSchema = true Code language: Kotlin (kotlin) As you see here, were not looping the notes list to find the row with the same dateAdded value as we had before, but were using only the updateNote.
Now, inside the NotesActivity, paste the following:
"notes_database"
}Code language: Kotlin (kotlin) private val noteDatabase by lazy { NoteDatabase.getDatabase(this).noteDao() }
}
@AmanGupta-ShOoTeR Did you get any solution for the comment above? In this tutorial, I will show you how to insert, read, update and delete data using Room through a simple notes app.
}
Inserting Data
For instance, if you want to update only 'title', you can reuse 'content' and 'photo' from already inserted data. val data = Intent() When we receive the edited note in the NotesActivity, were for looping the notes list to find the note that has the same dateAdded.