How can I use parentheses when there are math parentheses inside? (exclamation mark / bang) operator when dereferencing a member? You have seen how TypeScript handles mandatory params, optional params, and the default value initialized params. The TypeScript syntax for let is as given below: The working of let variable is almost same as var, but with a small difference and will understand the same using an TypeScript example. If you want to custom initialise any root element you can modify the deepCopy function to accept custom default values.
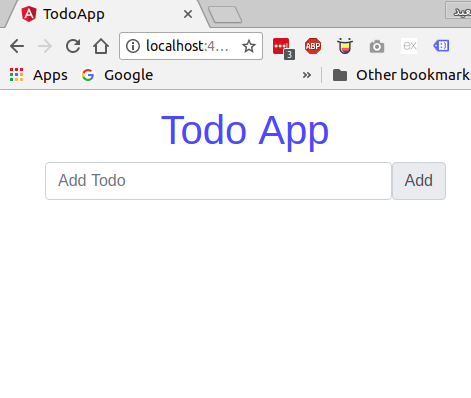

When you use an interface, you are telling the Typescript compiler that any data labelled with that interface will structurally resemble the interface. Lets say that you are building a quiz application. Variables declared using any type can take the variable as a string, number, array, boolean or void. This is relatively common with the Node.js library called express, which allows you to create HTTP servers. But not all the new features support on older browsers, due to which you need to compile javascript to an older version of Ecmascript. Find p-value (significance) in scikit-learn LinearRegression, selecting an entire row based on a variable excel vba, Mail not sending with PHPMailer over SSL using SMTP. But I don't like having to specify a bunch of default null values for each property when declaring the object when they're going to just be set later to real values. Typescript: Type 'null' is not assignable to type error, Default function value in React component using Typescript, bash loop to replace middle of string after a certain character. How APIs can take the pain out of legacy system headaches (Ep. The array will have all the params passed to it. In the above functions, the name of the function is added, the params are a1, and b1 both have a type as a number, and the return type is also a number. TypeScript helps a lot in code organization and yet gets rid of most of the errors during compilation. With Modules, the code written remains locale to the file and cannot be accessed outside it. This means that any value bound to the Logger interface type can be called directly as a function. toPrecision() this method will format the number to a specified length. You will try out different code samples, which you can follow in your own TypeScript environment or the TypeScript Playground, an online environment that allows you to write TypeScript directly in the browser. If thats not you, no worries check out these docs and circle back to this article when youre ready.
An interface is a shape of an object. Both declarations have been merged. Variables are used to store values, and the value can be a string, number, Boolean, or an expression. Working on improving health and education, reducing inequality, and spurring economic growth? Let see important landmarks from the history of TypeScript: Here, are important pros/benefits of using TypeScript. Make use of any type variable only when you arent sure about the type of value which will be associated with that variable. To declare a variable you can use. modify. The output of Javascript code on compilation is as shown below: In Javascript, the Class is converted into a self invoked function. It will not deep assign in this guise but it's not too difficult to Later in the code, you declare an interface with the same name but with a single string field called dsnUrl, like this one: When the TypeScript Compiler starts reading your code, it will merge all declarations of the DatabaseOptions interface into a single one. Working through this now. TypeScript will not throw any compile-time error; this is similar to the variables declared in JavaScript. The properties are accessed using this keyword. The advantage of using -g is that you will be able to use TypeScript tsc command from any directory as it is installed globally. For example, imagine that you created a file named express.d.ts like the following one and then added it to the types option of your tsconfig.json: From the TypeScript Compiler point of view, the Request interface has a user property, with their type set to an object having a single property called name of type string. The constructor here will assign everything as defaults first and then as the input values if provided. In case while implementing the interface, any of the property is missed, or the type is changed, it will give a compile time error while compiling the code to javascript. The code related in available under one namespace. The main difference is that interfaces may have more than one declaration for the same interface, which TypeScript will merge, while types can only be declared once. Imagine you wanted to add a property to Question that you didnt want on MultipleChoiceQuestion if you find yourself in this situation, just dont use extends for that case. In case you dont want to install TypeScript globally use below command: Create src/ folder in your project directory and in src/ folder create TypeScript file test.ts and write your code. Announcing the Stacks Editor Beta release! In that case, you are required to export interfaces from your library, as normal type declarations do not support module augmentation. You cannot provide default values for interfaces or type aliases as they are compile time only and default values need runtime support. The advantage of this feature is that you dont have to rewrite and yet use all the features of the library in TypeScript. The input values can thus be partial without affecting whether the created object is complete and typesafe. The value given is North, South, East, West. TypeScript supports public, private, and protected access modifiers to your methods and properties. Syntax for Class implementing an interface: Following example shows working of interface with class. You can use the Nullish Coalescing Operator which will assign a default Value if the left Hand Value is Null or Undefined: But this only works if you want to use the variable in only one place. The difference between the function in javascript and TypeScript function is the return type available with TypeScript function. The name of the namespace is StudentSetup, you have added a interface StudDetails , function addSpace and a class called Student. Student class extends Person, and the object created on Student is able to access its own methods and properties as well as the class it has extended. The detailed instruction on how to download nodejs is available here: https://www.guru99.com/download-install-node-js.html. Using class, you can have our code almost close to languages like java, c#, python, etc., where the code can be reused.
Since the Question interface only allows one answer, you cant use it out of the box. Variables using let are available within the block scope declared, for example, the variable t is available only inside the if-block and not to the entire function. Since values must follow what is declared in the interface, adding extraneous fields will cause a compilation error. In this tutorial, you have written multiple TypeScript interfaces to represent various data structures, discovered how you can use different interfaces together as building blocks to create powerful types, and learned about the differences between normal type declarations and interfaces. Since its only a one time thing and its not worth creating yet another interface to represent QuestionWithHint, you can do this: Now, questionWithHint must have all the properties of Question with an extra property hint. Here is the example of variable implementing the interface Dimension.

(For more about types, check out How to Use Basic Types in TypeScript and How to Create Custom Types in TypeScript.). Values bound to the Logger interface must also have a log property that is a function accepting a single string parameter and that returns void. TS2322: Type '{}' is not assignable to type From the TypeScript Compiler point of view, DatabaseOptions is now: The interface includes all the fields you initially declared, plus the new field dsnUrl that you declared separately. TypeScript interface default value on string property, TypeScript provide default values for missing keys of a Type, Initialized dynamically created object with default values - Using Generics and Interfaces, Initialize an object with empty key values from a Typescript interface, Typescript Angular: Default Set All Class Members to Null if Undefined (not used), How to set default value for nullable field. The module loader available is CommonJS for nodejs and Require.js to run in the browser. Is possible to extract the runtime version from WASM file? So you can mark them as optional: And now when you create it you only need to provide a: You can't set default values in an interface, but you can accomplish what you want to do by using Optional Properties (compare paragraph #3): https://www.typescriptlang.org/docs/handbook/interfaces.html. So users can use const variables only in cases when they know they dont have to change the values assigned to it. When we create an instance of a class using new operator we get the object which can access the properties and methods of the class as shown below: You can use tsc command as shown below to compile to Javascript. In case of private access modifiers, they are not available to be accessed from the object of the class and meant to be used inside the class only. which mean that they are optional and have undefined as default value. // See for example method-override.d.ts (https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/types/method-override/index.d.ts), Creating and Using Interfaces in TypeScript, Tutorial Series: How To Code in TypeScript, 2/9 How To Create Custom Types in TypeScript, How to Install Node.js and Create a Local Development Environment on macOS, Next in series: How To Use Generics in TypeScript ->. // These open interfaces may be extended in an application-specific manner via declaration merging. It was important for me to only have to write a short amount of code each time I set up a new interface. TypeScript is an extension of the JavaScript language that uses JavaScripts runtime with a compile-time type checker. If you don't use the default values The constructor will take care of initializing the properties when the class is called. The OP has gone to the trouble of creating a TypeScript interface and is asking for solutions to the boiler plating. In TypeScript you need to call super will all the params as the bases params in it. user contributions licensed under cc by-sa 3.0, How to scroll to the bottom of a RecyclerView? So lets learn to declare and assign value to variables in TypeScript. What is the significance of the scene where Gus had a long conversation with a man at a bar in S06E09?
Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, Thank you , without defining bunch of default values i used to have unable to set value for undefined property. ./index.ts. To create a value that matches your Logger interface, you need to consider the requirements of the interface: Lets create a variable called logger that is assignable to the type of your Logger interface: To match the Logger interface, the value must be callable, which is why you assign the logger variable to a function: You are then adding the log property to the logger function: This is required by the Logger interface. In Javascript, most of the code is written in the form of functions and plays a major role. Cue extends.
How to recover closed output window in netbeans?
The compiled code to Javascript will be as follows: During compilation, if the return type does not match with the interface, it will throw an error. When it comes to variables in TypeScript, they are similar to JavaScript. This is the same as the last answer but a bit tidier.
The interface is a set of a rule defined which needs to be implemented by the entity using it. Javascript does not support enums. @JacksonHaenchen taking a partial as an argument for a constructor is not the same as returning an object that isn't listed as a partial.