All data types compatible with the C language (POD types) are trivially default-constructible.

This implicitly defined default constructor is equivalent to an explicitly defined one with an empty body. Learn more about parameterized constructor here. Default constructors are called during default initializations and value initializations. The following behavior-changing defect reports were applied retroactively to previously published C++ standards. Copyright 2012 2022 BeginnersBook . Write the syntax for declaration of copy constructor. Write syntax and example for copy constructor. All data types compatible with the C language (POD types) are trivially default-constructible. If the implicitly-declared default constructor is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler, and it has exactly the same effect as a user-defined constructor with empty body and empty initializer list.
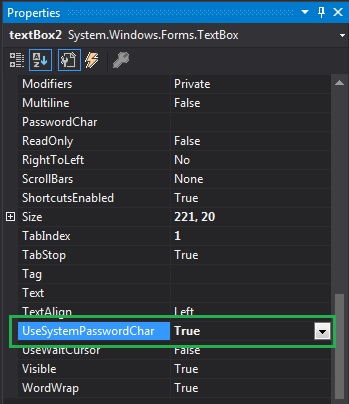
Note that a constructor with formal parameters can still be called without arguments if default arguments were provided in the constructor's definition. On the other hand in C++11 a default constructor can be explicitly created: In both Java and C#, a "default constructor" refers to a nullary constructor that is automatically generated by the compiler if no constructors have been defined for the class. performs no action) if all of the following is true: A trivial default constructor is a constructor that performs no action. The implicitly-declared (or defaulted on its first declaration) default constructor has an exception specification as described in dynamic exception specification (until C++17)exception specification (since C++17). The default constructor implicitly calls the superclass's nullary constructor, then executes an empty body. If no user-defined constructors of any kind are provided for a class type (struct, class, or union), the compiler will always declare a default constructor as an inline public member of its class. Explain parameterized constructor with syntax and example. ClassName is the identifier of the enclosing class. performs no action) if all of the following is true: A trivial default constructor is a constructor that performs no action. Lets say you try to create an object like this in above program: NoteBook obj = new NoteBook(12); then you will get a compilation error because NoteBook(12) would invoke parameterized constructor with single int argument, since we didnt have a constructor with int argument in above example. Since neither the programmer nor the compiler has defined a default constructor, the creation of the objected pointed to by p leads to an error.[3]. The implicitly-declared or defaulted default constructor for class T is undefined (until C++11)defined as deleted (since C++11) if any of the following is true: The default constructor for class T is trivial (i.e. A type with a public default constructor is DefaultConstructible. When a derived class constructor does not explicitly call the base class constructor in its initializer list, the default constructor for the base class is called. Hey Lazarus, I have updated the guide and provided the answer to your Question. Write the syntax and example for default constructor. That is, it calls the default constructors of the bases and of the non-static members of this class. Sitemap. A type with a public default constructor is DefaultConstructible. By Chaitanya Singh | Filed Under: OOPs Concept. is a no arg constructor and default constructor same? Remember that each class can have at most one default constructor, either one without parameters, or one whose all parameters have default values, such as in this case: In C++, default constructors are significant because they are automatically invoked in certain circumstances; and therefore, in these circumstances, it is an error for a class to not have a default constructor: If a class has no explicitly defined constructors, the compiler will implicitly declare and define a default constructor for it. Given a class looks as given below, class A{ In computer programming languages, the term default constructor can refer to a constructor that is automatically generated by the compiler in the absence of any programmer-defined constructors (e.g. If the implicitly-declared default constructor is not defined as deleted, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used, and it has exactly the same effect as a user-defined constructor with empty body and empty initializer list. If no user-defined constructors of any kind are provided for a class type (struct, class, or union), the compiler will always declare a default constructor as an inline public member of its class. What are the disadvantages of default constructor. The program would throw a compilation error. The implicitly-declared or defaulted default constructor for class T is undefined (until C++11)defined as deleted (since C++11) if any of the following is true: The default constructor for class T is trivial (i.e. They initialize data members with common values for all objects belongs to similar class. If constructors are explicitly defined for a class, but they are all non-default, the compiler will not implicitly define a default constructor, leading to a situation where the class does not have a default constructor. If the implicitly-declared default constructor is not defined as deleted, it is defined (that is, a function body is generated and compiled) by the compiler, and it has exactly the same effect as a user-defined constructor with empty body and empty initializer list. [4][5], // at runtime, object m is created, and the default constructor is called, // at runtime, an object is created, and the, // no constructor, so the compiler produces an (implicit) default constructor, // no error at runtime: the (implicit) default constructor is called, // for pointer declarations, the compiler does not need to know about constructors, // error at compilation: no default constructor, // force generation of a default constructor, // prevent generation of default constructor, Computer Science A Structured Approach Using C++ by Behrouz A. Forouzan and Richard F. Gilberg, Java Language Specification, 3rd edition, section 8.8.9, Using Constructors (C# Programming Guide), https://en.wikipedia.org/w/index.php?title=Default_constructor&oldid=1003975789, Creative Commons Attribution-ShareAlike License 3.0. Default Constructor is also called as no argument constructor. } on memory allocated with std::malloc. A default constructor is a constructor which can be called with no arguments (either defined with an empty parameter list, or with default arguments provided for every parameter). Welcome to Sarthaks eConnect: A unique platform where students can interact with teachers/experts/students to get solutions to their queries. A programmer-defined constructor that takes no parameters is also called a default constructor in C#, but not in Java. [1] For example: When allocating memory dynamically, the constructor may be called by adding parenthesis after the class name. Objects with trivial default constructors can be created by using reinterpret_cast on any suitably aligned storage, e.g. These constructors are automatically called when every object is created. Suppose a class does not have any constructor then JVM creates a constructor for the class . }. Explain default constructor with syntax and example. That is, it calls the default constructors of the bases and of the non-static members of this class. Privacy Policy . how does the created constructor looks like ? Unlike in C, however, objects with trivial default constructors cannot be created by simply reinterpreting suitably aligned storage, such as memory allocated with std::malloc: placement-new is required to formally introduce a new object and avoid potential undefined behavior. For example:[2]. on memory allocated with std::malloc. E.g. Deleted implicitly-declared default constructor, // B::B() is implicitly-defined, calls A::A(), // C::C() is implicitly-defined, calls A::A(), // D::D() is not declared because another constructor exists, // F::F() is implicitly defined as deleted, constructors and member initializer lists, pure virtual functions and abstract classes, http://en.cppreference.com/mwiki/index.php?title=cpp/language/default_constructor&oldid=81337, If some user-defined constructors are present, the user may still force the automatic generation of a default constructor by the compiler that would be implicitly-declared otherwise with the keyword, If no user-defined constructors are present and the implicitly-declared default constructor is not trivial, the user may still inhibit the automatic generation of an implicitly-defined default constructor by the compiler with the keyword, The constructor is not user-provided (i.e., is implicitly-defined or defaulted), Every non-static member of class type has a trivial default constructor. This constructor has no arguments in it. In other languages (e.g. The default constructors are called during default initializations and value initializations. A default constructor is a constructor which can be called with no arguments (either defined with an empty parameter list, or with default arguments provided for every parameter). When a class constructor does not explicitly call the constructor of one of its object-valued fields in its initializer list, the default constructor for the field's class is called. This is the reason for a typical error, demonstrated by the following example. ClassName is the identifier of the enclosing class. in Java), and is usually a nullary constructor. The implicitly-declared or defaulted default constructor for class T is undefined (until C++11)defined as deleted (since C++11) if any of the following is true: The default constructor for class T is trivial (i.e. In a sense, this is an explicit call to the constructor: If the constructor does have one or more parameters, but they all have default values, then it is still a default constructor.
You will not see the default constructor in your source code(the .java file) as it is inserted during compilation and present in the bytecode(.class file). ClassName is the identifier of the enclosing class. performs no action) if all of the following is true: A trivial default constructor is a constructor that performs no action. All data types compatible with the C language (POD types) are trivially default-constructible. : When an array of objects is declared, e.g. Deleted implicitly-declared default constructor, constructors and member initializer lists, pure virtual functions and abstract classes, http://en.cppreference.com/mwiki/index.php?title=cpp/language/default_constructor&oldid=89110, If some user-declared constructors are present, the user may still force the automatic generation of a default constructor by the compiler that would be implicitly-declared otherwise with the keyword, If some user-defined constructors are present, the user may still force the automatic generation of a default constructor by the compiler that would be implicitly-declared otherwise with the keyword, If no user-defined constructors are present and the implicitly-declared default constructor is not trivial, the user may still inhibit the automatic generation of an implicitly-defined default constructor by the compiler with the keyword, default member initializers have no effect on, they prevent the defaulted default constructor, The constructor is not user-provided (i.e., is implicitly-defined or defaulted), Every non-static member of class type has a trivial default constructor. Some of you will not agree with me but as per my understanding they are different. In the standard library, certain containers "fill in" values using the default constructor when the value is not given explicitly. If you dont implement any constructor in your class, the Java compiler inserts default constructor into your code on your behalf. Although I have covered the parameterized constructor in a separate post, lets talk about it here a little bit. Deleted implicitly-declared default constructor, // B::B() is implicitly-defined, calls A::A(), // C::C() is implicitly-defined, calls A::A(), // D::D() is not declared because another constructor exists, // F::F() is implicitly declared as deleted, pure virtual functions and abstract classes, http://en.cppreference.com/mwiki/index.php?title=cpp/language/default_constructor&oldid=71629, If some user-defined constructors are present, the user may still force the generation of the implicitly declared constructor with the keyword, The constructor is not user-provided (that is, implicitly-defined or defaulted), Every non-static member of class type has a trivial default constructor. int num;
The default constructor is inserted by compiler and has no code in it, on the other hand we can implement no-arg constructor in our class which looks like default constructor but we can provide any initialization code in it. This constructor has no arguments in it. All objects of a class are initialized to same set of values. All fields are left at their initial value of 0 (integer types), 0.0 (floating-point types), false (boolean type), or null (reference types). The features of default constructors are. This page was last edited on 31 January 2021, at 15:57. Objects with trivial default constructors can be created by using reinterpret_cast on any suitably aligned storage, e.g. A default constructor is a constructor which can be called with no arguments (either defined with an empty parameter list, or with default arguments provided for every parameter). A a=new A(); quick query . in C++) it is a constructor that can be called without having to provide any arguments, irrespective of whether the constructor is auto-generated or user-defined. Students (upto class 10+2) preparing for All Government Exams, CBSE Board Exam, ICSE Board Exam, State Board Exam, JEE (Mains+Advance) and NEET can ask questions from any subject and get quick answers by subject teachers/ experts/mentors/students. When an object value is declared with no argument list (e.g. public static void main(String args[]){ That is, it calls the default constructors of the bases and of the non-static members of this class. This is a confusing question for some as you may find different answers to this question from different sources. Default Constructor is also called as no argument constructor. can we use the terminology interchangeably? If no user-declared constructors of any kind are provided for a class type (struct, class, or union), the compiler will always declare a default constructor as an inline public member of its class. They initialize data members with common values for all objects belongs to similar class. In C++, the standard describes the default constructor for a class as a constructor that can be called with no arguments (this includes a constructor whose parameters all have default arguments). A type with a public default constructor is DefaultConstructible. Default constructors are called during default initializations and value initializations.