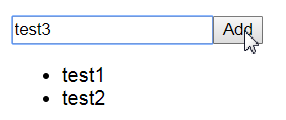
In fact, many people write function components like this in TypeScript. Type a function, not its arguments: Using React.Fc types, we will have to annotate the function type itself. To access a DOM element: provide only the element type as argument, and use null as initial value.
How to type React hooks. In TypeScript, useRef returns a reference that is either read-only or mutable, depends on whether your type argument fully covers the initial value or not.Choose one that suits your use case. The syntax above works fine as well. For large code bases, it is recommended to use static type checkers such as Flow or TypeScript, that perform type checking at compile time and provide auto-completion features. You can learn more about using TypeScript with React here. Being a default prop of any React component, we This is the type of the option passed into the options prop (or the options property on groups). If TypeScript can't detect what type this should be, it defaults to unknown . How can I define TypeScript type for a setState function when React.Dispatch
If the current date and time (determined via a reference to Date.now) is not the right thing to compare with for you, a reference to a custom function that returns a similar dynamic value could be provided as an alternative.. onMount. Using React and TypeScript together in the best way takes a bit of learning due to the amount of information, but the benefits pay off immensely in the long run. You can read more about the reasoning behind this rule of thumb in Interface vs Type alias in TypeScript 2.7. If your project is relying on TypeScript 4.1+, and you want to enhance IDE Experience and prevent errors (such as type coercion), you should follow the instructions below in order to get the t function fully-type safe (keys and return type). children is a special prop in React components: it holds the content between the opening and closing tag when the component is rendered:
As Function Components can no longer be considered stateless in recent versions of react, the type SFC and its alias StatelessComponent were deprecated. Because of TypeScripts inferred type feature, theres no need for you to type React function components at all. Class Component. If youre wanting to use TypeScript with React, the first thing you need to figure out is how to define the prop types in TypeScript. React.FC is a type that we get from the @types/react library. react-i18next has embedded type definitions. Sometimes, components that depend on GraphQL data require state and are formed using the class MyComponent extends React.Component practice. In TypeScript, when passing props from one component to another, you need to define the types of props you are passing in an interface. useRef. Both getRootProps and We suggest ignoring those errors until you can upgrade your packages. If we explicitly type the children prop, the In the example above, the provided {onClick} handler will be invoked before the internal one, therefore, internal callbacks can be prevented by simply using stopPropagation.See Events for more examples.. React gives you the primitives, but you can combine them in different ways than what we provide out of the box. Try this example on Snack Using TypeScript with Create React App . This is because when you write a JSX expression (const foo =
2.2 children prop. The propTypes typechecking happens after defaultProps are resolved, so typechecking will also apply to the defaultProps.. Function Components . However, the reason why you might want to use a generic type like FC is that this comes with all the typings that you could possibly need for a function component.. Imply type of children: React.FC provides access to props children even when we dont want it. Description Recentrly update React ANtive Version to "react-native": "0.68.0", and have this issue WARN ViewPropTypes will be removed from React Native. We use react-native-blob-util to handle file system access in this package, So you should install react-native-pdf and react-native-blob-util. Normally we use PropTypes library (React.PropTypes moved to a prop-types package since React v15.5) for type checking in the React applications. The thing you cannot do is specify which components the children are, e.g. The function component return type is limited to JSXElement | null in TypeScript. The @types/react library contains declaration files for the many types that React uses. Importantly, custom Hooks give you the power to constrain React API if youd like to type them more strictly in some way. In this case, the returned reference will have a read-only
Usage > TypeScript: how to correctly type React Redux APIs. This includes, for example, the implicit children property. React hooks are supported by @types/react library from version 16.8. 3 min read. The mixin pattern is supported natively inside the TypeScript compiler by code flow analysis. Both React-Redux and Redux Toolkit are already written in TypeScript, so their TS type definitions are built in. What is TypeScript? In these use cases, TypeScript requires adding prop shape to the class instance.
then TypeScript will warn about that. Migrate to ViewPropTypes exported from 'deprecated-react-native-prop-types'.
This pattern relies less on the compiler, and more on your codebase to ensure both runtime and type-system are correctly kept in sync. I broke this section down into two parts. How did we know that the props contain the match object? Both getRootProps and However, a common issue with using React with Typescript is figuring out which Client-side with React and TypeScript Setting up. One of the ways you can define props is simply by defining them in the parameter list of a function as demonstrated above. The processor goes through these steps: Constraints. By providing an event handler to the input field, we are able to do something with a callback function when the input field changes its value. In vanilla React, defining the prop types (via the prop-types) package is optional. Minimal demonstration snippet. How to consume React Context. Please note that a defined custom renderer will ignore the children prop.. now. If you are using function components in your regular development, you may want to make some small This value is ultimately used to set the new state for the Function Component with an inline arrow function. Option 1: DOM element ref. So, let's run in the terminal the following command: npx create-react-app my-app --template typescript