Specifically, the most derived function that is not more derived than the current constructor or destructor's class is called. In manual memory management contexts, the situation can be more complex, particularly in relation to static dispatch. for the purpose of avoiding conflicts. What are the guidelines of using namespaces? Thank you for reading this article and please check out the other articles provided by LinuxHint.com. Even if a function is specified as pure virtual, it can be given a default implementation. The derived class Status is defined to get the student percentage by accessing the Parent class as an interface. We will be doing some mathematical calculations in the pure virtual method. Classes Python 3.9.2 documentation", "Writing Final Classes and Methods (The Java Tutorials > Learning the Java Language > Interfaces and Inheritance)", Pure virtual destructors - cppreference.com, "abc Abstract Base Classes: @abc.abstractmethod", "N4659: Working Draft, Standard for Programming Language C++", "Never Call Virtual Functions during Construction or Destruction", "C++ corner case: You can implement pure virtual functions in the base class", "Joy of Programming: Calling Virtual Functions from Constructors", https://en.wikipedia.org/w/index.php?title=Virtual_function&oldid=1096837211, Articles needing additional references from March 2013, All articles needing additional references, Articles with unsourced statements from October 2021, Creative Commons Attribution-ShareAlike License 3.0, This page was last edited on 6 July 2022, at 23:40. doOperation(). For example, if an object of type Wolf that inherits Animal is created, and both have custom destructors, the one called will be the one declared in Wolf. Classes containing pure virtual methods are termed "abstract" and they cannot be instantiated directly. However, because many OOP languages do not support multiple inheritance, they often provide a separate interface mechanism. In C, the mechanism behind virtual functions could be provided in the following manner: A pure virtual function or pure virtual method is a virtual function that is required to be implemented by a derived class if the derived class is not abstract. What is a namespace? What are the guidelines of using namespaces. After saving the C++ pure virtual code, compile it with the G++ compiler package. [10] A conforming C++ implementation is not required (and generally not able) to detect indirect calls to pure virtual functions at compile time or link time. In such a use, derived classes will supply all implementations. This object is used to call the getscore() method to acquire marks from the user. After running the file, we have got the following output: The result of this illustration can be analyzed in the attached image above. If most or all implementations of the pure virtual function will contain duplicate code, that code can instead be moved to the base class version, making the code easier to maintain. This class contains a protected integer type variable named score used to get marks from the user. The same object is used to call the percentage() function to calculate the percentage of marks entered by the user. If there are base class methods overridden by the derived class, the method actually called by such a reference or pointer can be bound (linked) either 'early' (by the compiler), according to the declared type of the pointer or reference, or 'late' (i.e., by the runtime system of the language), according to the actual type of the object is referred to. This virtual function can be used in the abstract class. [7]:13.4.6[8] This is true even if the class contains an implementation for that pure virtual function, since a call to a pure virtual function must be explicitly qualified. Get monthly updates about new articles, cheatsheets, and tricks. There are a couple of reasons why we might want to do this: If we want to create a class that can't itself be instantiated, but doesn't prevent its derived classes from being instantiated, we can declare the destructor as pure virtual. 1309 S Mary Ave Suite 210, Sunnyvale, CA 94087
Virtual functions are resolved 'late'. As the Parent class is abstract, we cannot create its object. In short, a virtual function defines a target function to be executed, but the target might not be known at compile time. The pointer *p of Parent class has been accessing the address of Child class object c. Despite this, the function will still be considered abstract, and derived classes will have to define it before they can be instantiated. Eat cannot know which function (if any) to call at compile time. Thus, this article is implemented to determine how the pure virtual function can be stated in the base class and defined in the derived class. Add the header package of the input-output stream and use the standard namespace in the code, as well. Object-oriented languages typically manage memory allocation and de-allocation automatically when objects are created and destroyed. /* which contains the virtual function Animal.Eat */, /* Since Animal.Move is not a virtual function. The Child class Status contains the definition of a pure virtual method percentage() in it: The pure virtual function percentage() is calculating the percentage of student marks. Finally, we have used the examples of using a pure virtual function in the C++ abstract class.
So, start by opening the same file and modify it a little. The G++ compiler has been used for the implementation of examples. C++ and Python) are permitted to contain an implementation in their declaring class, providing fallback or default behaviour that a derived class can delegate to, if appropriate.[5][6]. Most programming languages, such as JavaScript, PHP and Python, treat all methods as virtual by default[1][2] and do not provide a modifier to change this behavior. This percentage is found with the help of a percentage formula using the marks of a student. We have used abstract and derived classes to illustrate the concept of pure virtual function.
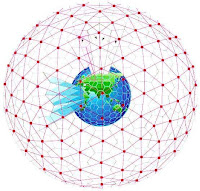
And as the destructor is most likely already virtual to prevent memory leaks during polymorphic use, we won't incur an unnecessary performance hit from declaring another function virtual.
This can be useful when making interfaces. Then, the pure virtual function percentage() is declared to 0 here. Languages differ in their behavior while the constructor or destructor of an object is running. Note: The operating system used in this tutorial is Ubuntu 20.04. What happens when two namespaces having the same name?
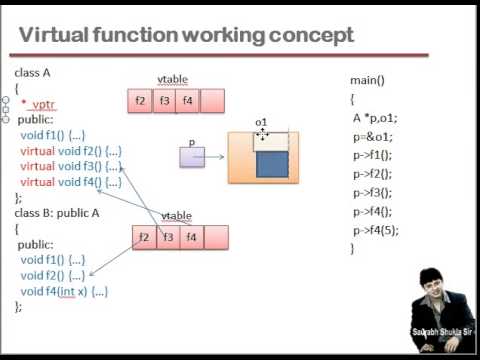
Then the pointer *p is calling the pure virtual function show() by a reference. The Child class contains the definition of a show() method. We have declared an abstract class named Result after the namespace. In this case, the derived class' version of the function is even allowed to call the base class' version. A pure virtual function in the C++ programming language is a widely known concept in C++ inheritance. The cout clause in this method requires the user to enter some score. Being the destructor, it must be defined anyways, if we want to be able to deallocate the instance. For example, a base class Animal could have a virtual function Eat. A simple public type method has been defined in the Abstract class named getscore(). // The non virtual function Move is inherited but not overridden. - To name a namespace, utilize the company name followed by the department and optionally followed by the features and technology used. What is name lookup? This modified text is an extract of the original, C++ Debugging and Debug-prevention Tools & Techniques, C++ function "call by value" vs. "call by reference", Curiously Recurring Template Pattern (CRTP), RAII: Resource Acquisition Is Initialization, SFINAE (Substitution Failure Is Not An Error), Side by Side Comparisons of classic C++ examples solved via C++ vs C++11 vs C++14 vs C++17, std::function: To wrap any element that is callable, Behaviour of virtual functions in constructors and destructors, Using override with virtual in C++11 and later, as the destructor is most likely already virtual to prevent memory leaks during polymorphic use. We can also specify that a virtual function is pure virtual (abstract), by appending = 0 to the declaration. In such a design pattern, the abstract class which serves as an interface will contain only pure virtual functions, but no data members or ordinary methods. Inheritable and overridable function or method for which dynamic dispatch is facilitated, Abstract classes and pure virtual functions, Behavior during construction and destruction. Here comes the main() method. This Child class has been accessing the Parent class while using it as an interface. [11] Some design patterns, such as the Abstract Factory Pattern, actively promote this usage in languages supporting this ability. Based on the information provided, we believe that it will be easy for you to work on pure virtual functions from now on. Lets look at another example of using a pure virtual function in C++. If the language in question uses automatic memory management, the custom destructor (generally called a finalizer in this context) that is called is certain to be the appropriate one for the object in question. [citation needed]. Overloading occurs when two or more methods in one class have the same method name but different parameters. - A conceptual space for grouping identifiers, classes etc. The declaration value for the pure virtual function is 0. The main() function contains the object creation of the Child class. If it is not 'virtual', the method is resolved 'early' and selected according to the declared type of the pointer or reference. In Java and C#, the derived implementation is called, but some fields are not yet initialized by the derived constructor (although they are initialized to their default zero values). We are starting with a simple example to illustrate the overall working and structure of a pure virtual function in the C++ abstract class. */, /* implementation of Llama.Eat this is the target function, to be called by 'void Eat(struct Animal *).' After coming back to the terminal, compile the code first with the g++ compiler package. This allows a programmer to process a list of objects of class Animal, telling each in turn to eat (by calling Eat), without needing to know what kind of animal may be in the list, how each animal eats, or what the complete set of possible animal types might be. If the function in question is 'virtual' in the base class, the most-derived class's implementation of the function is called according to the actual type of the object referred to, regardless of the declared type of the pointer or reference. Classes with one or more pure virtual functions are considered to be abstract, and cannot be instantiated; only derived classes which define, or inherit definitions for, all pure virtual functions can be instantiated. Some runtime systems will issue a pure virtual function call error when encountering a call to a pure virtual function at run time. [7]:15.7.3[8][9] If that function is a pure virtual function, then undefined behavior occurs. The concept of the virtual function solves the following problem: In object-oriented programming, when a derived class inherits from a base class, an object of the derived class may be referred to via a pointer or reference of the base class type instead of the derived class type. */, // base class does not implement Animal.Eat, /* init objects as instance of its class */, // cannot resolve Animal.Eat so print "Not Implemented" to stderr, Learn how and when to remove this template message, "Polymorphism (The Java Tutorials > Learning the Java Language > Interfaces and Inheritance)", "9. // The class "Animal" may possess a definition for Eat if desired. virtual type func-name(parameter-list) = 0; What is a namespace? However, some languages provide modifiers to prevent methods from being overridden by derived classes (such as the final keyword in Java[3] and PHP[4]). However, some object-oriented languages allow a custom destructor method to be implemented, if desired. Virtual functions allow a program to call methods that don't necessarily even exist at the moment the code is compiled. Subclass Llama would implement Eat differently than subclass Wolf, but one can invoke Eat on any class instance referred to as Animal, and get the Eat behavior of the specific subclass. You have to know that an abstract class doesnt occupy any object, i.e., not instantiated. However, we can use pointers or references to access this class and its functions. We have added three different marks at every execution and got three different percentages every time. Although pure virtual methods typically have no implementation in the class that declares them, pure virtual methods in some languages (e.g. Overriding means having two methods with the same method name and parameters. The show() method has been displaying some text with the cout clause. Implementing doOperation() would not make sense in the MathSymbol class, as MathSymbol is an abstract concept whose behaviour is defined solely for each given kind (subclass) of MathSymbol. In C++, using such purely abstract classes as interfaces works because C++ supports multiple inheritance. Animal.Eat can only be resolved at run time when Eat is called. All the work should start from here: At the start of a main() method, the Parent class pointer *p has been created. The functions would get the marks from the user as input via the cin clause. You can use other Linux distros or Windows systems. Hello, I am a freelance writer and usually write for Linux and other technology related content, Linux Hint LLC, [emailprotected]
The Child class has been initiated with the object c. And even if methods owned by the base class call the virtual method, they will instead be calling the derived method. It contains a single pure virtual function declaration called show(). The derived class named Child has been initialized. After that, run the file with the a.out query. /* unlike Move, which executes Animal.Move directly. Every C++ code starts with the input-output stream header included in it. Similarly, a given subclass of MathSymbol would not be complete without an implementation of For this reason, calling virtual functions in constructors is generally discouraged. Pure virtual methods typically have a declaration (signature) and no definition (implementation). If an object of type Wolf is created but pointed to by an Animal pointer, and it is this Animal pointer type that is deleted, the destructor called may actually be the one defined for Animal and not the one for Wolf, unless the destructor is virtual. This concept is an important part of the (runtime) polymorphism portion of object-oriented programming (OOP). The namespace standard must be declared after that. An abstract class has been initialized named Parent. A subclass of an abstract class can only be instantiated directly if all inherited pure virtual methods have been implemented by that class or a parent class. In C++, virtual methods are declared by prepending the virtual keyword to the function's declaration in the base class. As an example, an abstract base class MathSymbol may provide a pure virtual function doOperation(), and derived classes Plus and Minus implement doOperation() to provide concrete implementations. The marks have been obtained by the variable score having marks entered by the user. It will request you to enter your marks. Lets save the code first and exit the file by using Ctrl+S and Ctrl+S, respectively. - A name lookup is a name in the specified logically grouped names in the namespace © Copyright 2016. Pure virtual functions can also be used where the method declarations are being used to define an interface - similar to what the interface keyword in Java explicitly specifies. All Rights Reserved. So, create a new C++ file with the help of the touch query and open it with an editor, such as Nano editor. Privacy Policy and Terms of Use. Overloading is also referred to as function matching, and overriding as dynamic function mapping. In object-oriented programming, in languages such as C++, and Object Pascal, a virtual function or virtual method is an inheritable and overridable function or method for which dynamic dispatch is facilitated. This pure function has been initialized with 0. This is particularly the case with C++, where the behavior is a common source of programming errors if destructors are not virtual. In C++, the "base" function is called. This modifier is inherited by all implementations of that method in derived classes, meaning that they can continue to over-ride each other and be late-bound. It can only be stated in the abstract class, and it cannot be defined. An example is the Java programming language.
No errors have been found so far.